Best development practices to grow as a programmer.
Here you can find out this article in spanish
Excellent development practices that can help you maintain clean, organized, and easily maintainable code
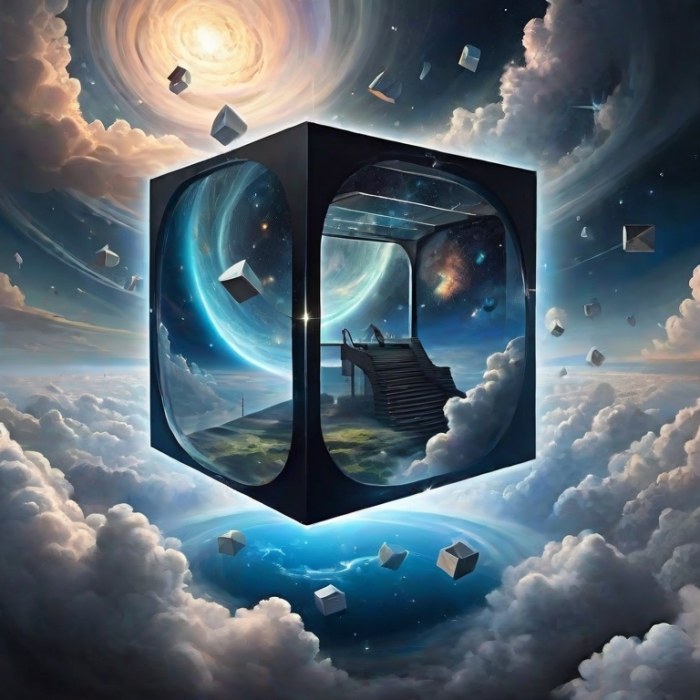
Let's start thinking outside of the box
-
Avoid leaving unused code whenever possible:
// Avoid leaving unused code like this // const unusedVariable = "I'm not used";
-
Comment your code when it's very extensive and/or complex to understand:
// Commenting on complex code can help other developers understand the logic if (condition) { // Complex logic here... }
-
Use version control software (GIT): Version control systems like Git allow you to track changes and collaborate with others.
-
Do not version commented-out or erroneous code:
// This commented-out code should not be in version control // const obsoleteCode = "I'm not needed anymore";
-
When making a commit, provide a descriptive comment of what is being versioned:
git commit -m "feat: Add new feature to the project"
-
When working with classes/components, use camelCase naming:
class myComponent { // Class name in camelCase }
-
When working with methods, attributes, and variables, use names in lowerCamelCase format:
const myVariableName = 'example' // Variable name in lowerCamelCase
-
When working with constants, use descriptive names in uppercase separated by underscores if necessary:
const IMPORTANT_CONSTANT = 42 // Descriptive constant name in uppercase with underscores
-
If paths for URL(s) are required, use descriptive lowercase names separated by hyphens:
<a href="/my-descriptive-url">Link</a> <!-- Descriptive URL with hyphens -->
-
Associate a component with a single view whenever possible:
// A component associated with a single view
- When dealing with associated resources, use descriptive names referencing their functionality:
const homeScreenImage = 'image.jpg' // Descriptive resource name referencing its use
- To validate functionality, methods, APIs, libraries, etc., use a branch named "Test":
git checkout -b test/feature-name // Creating a test branch for validation
Estos ejemplos ilustran cómo aplicar cada una de las prácticas mencionadas en tu lista.